hXXX.com
Some of my Projects
Explore my categorized projects below. The math section includes coding projects like a calculator, and the science section has a density calculator.
- All Projects
- Math
- LeetCode
- HackerRank
- Python
- Science
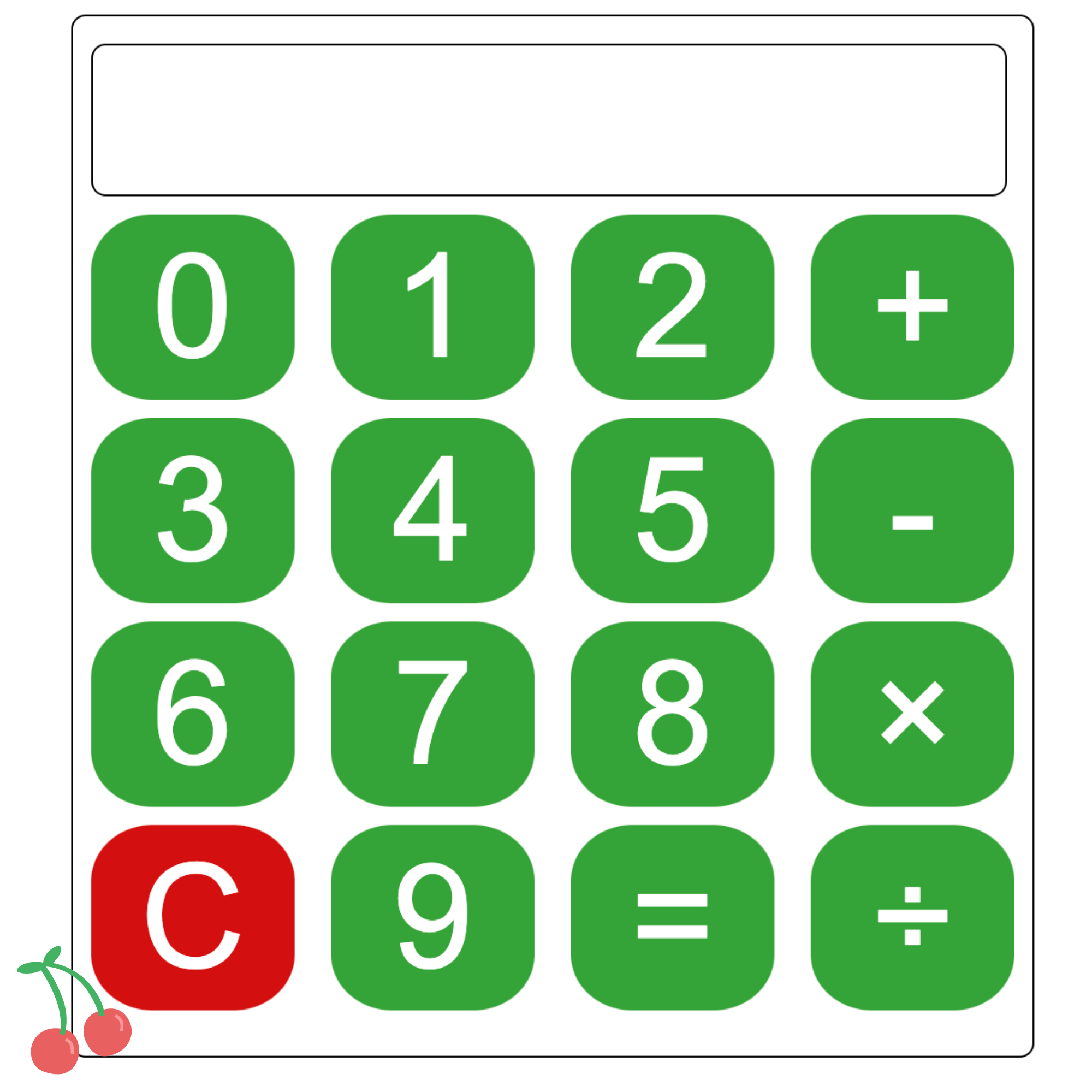
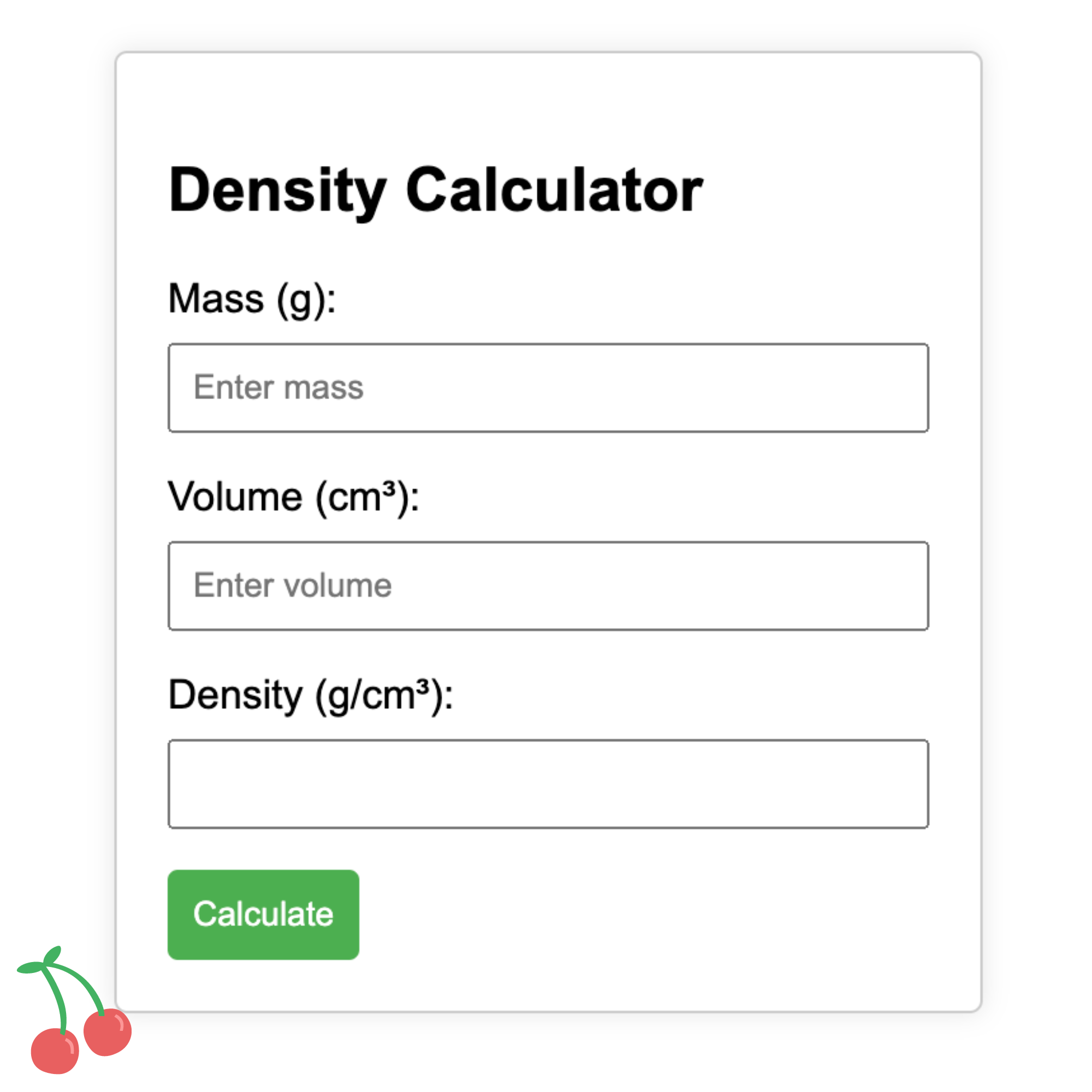
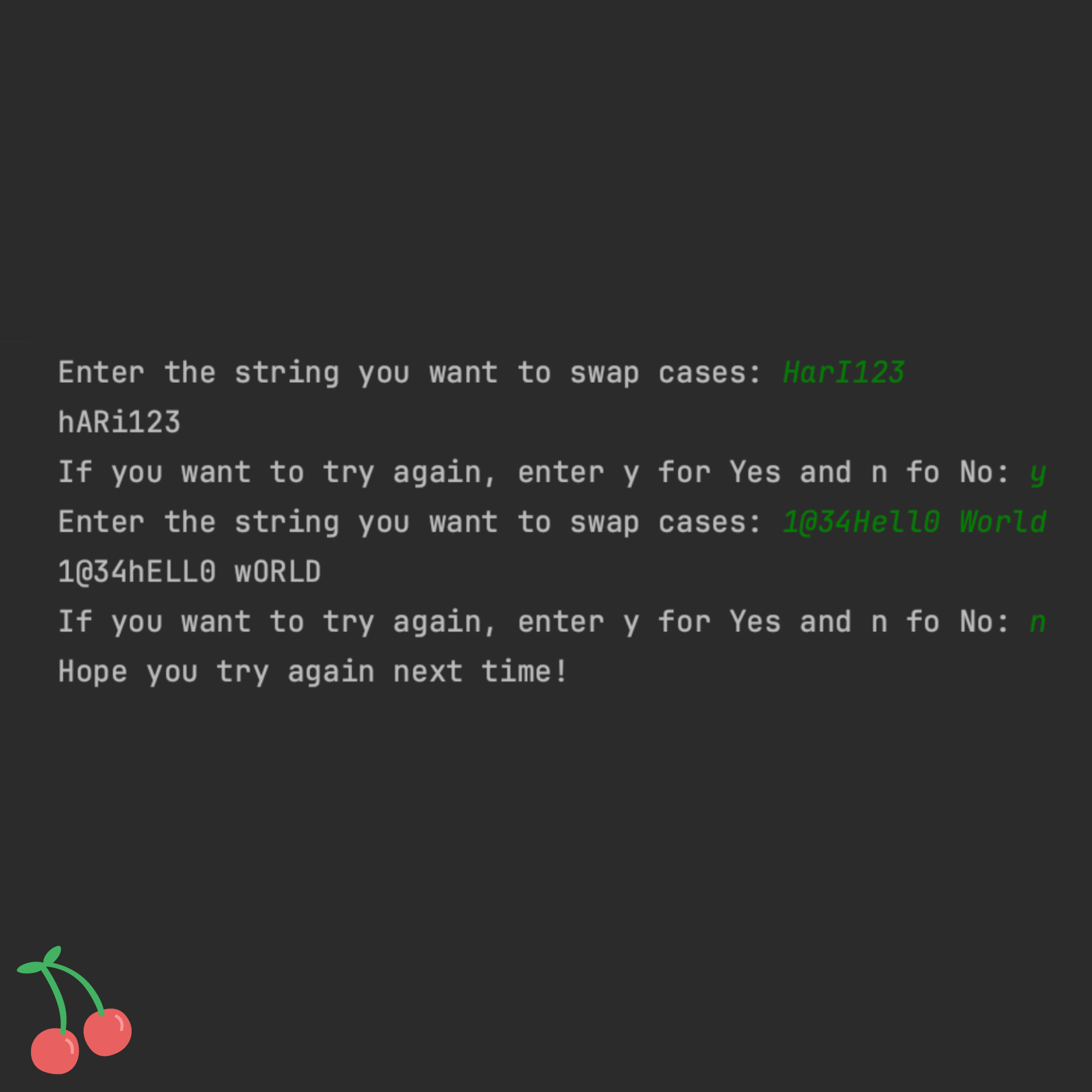
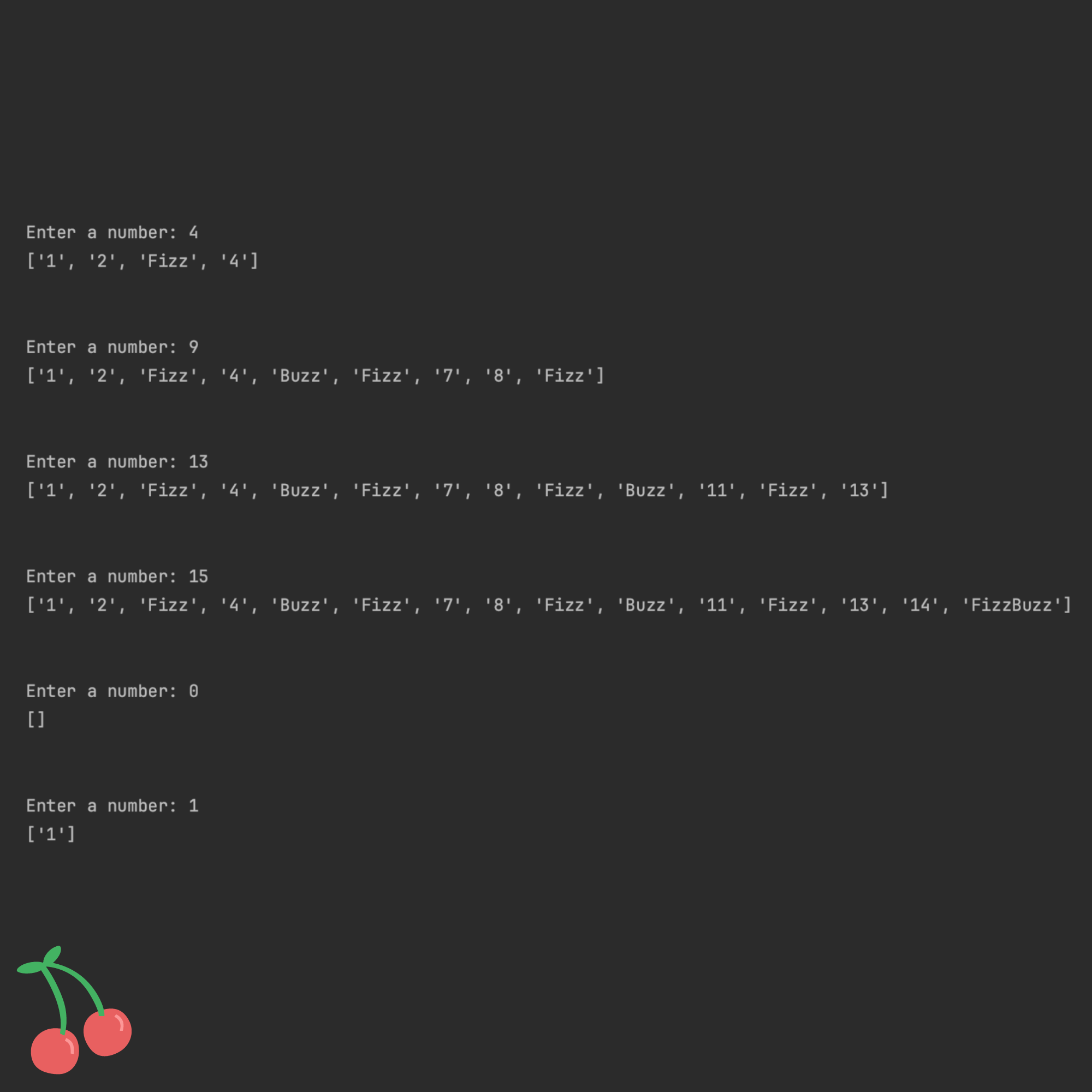
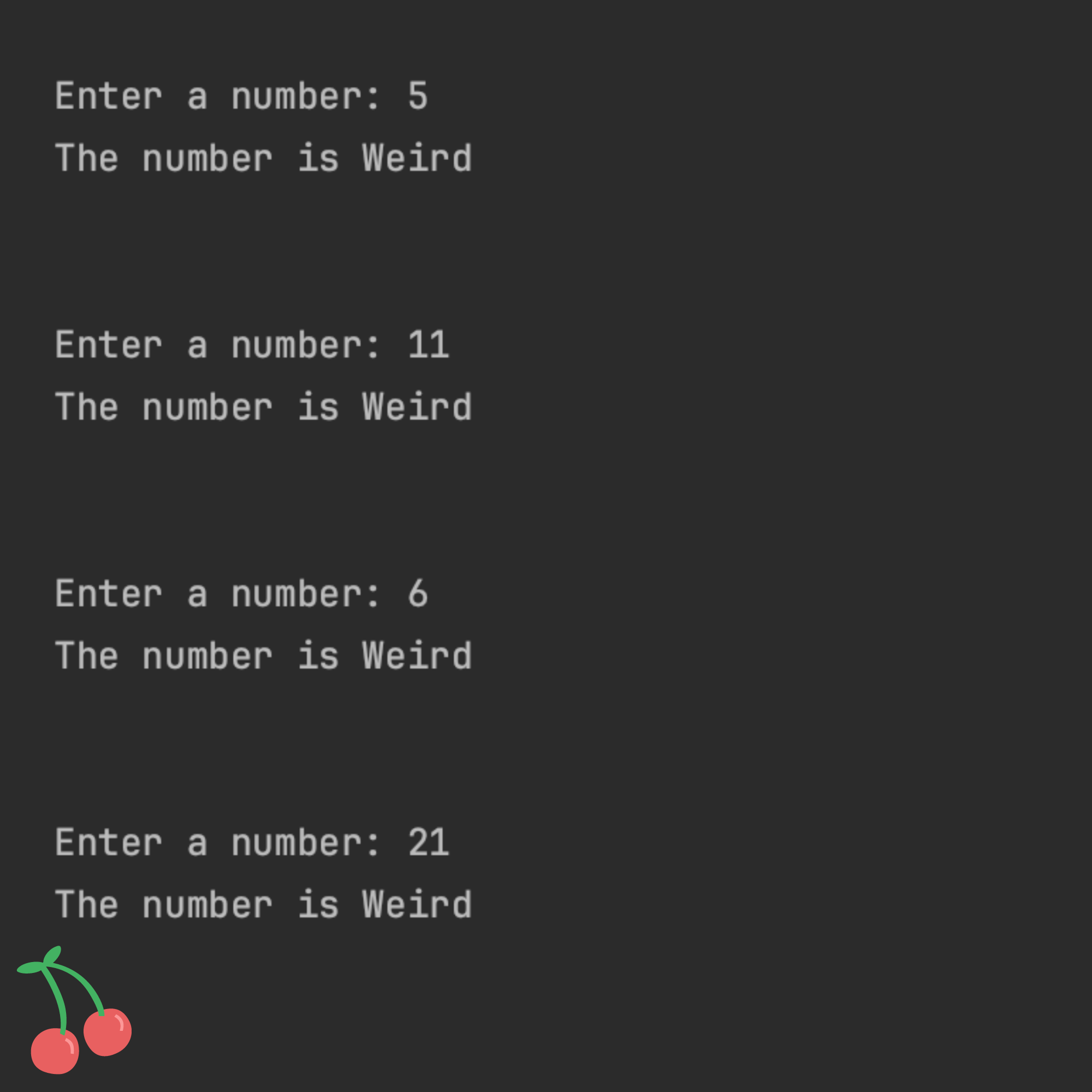
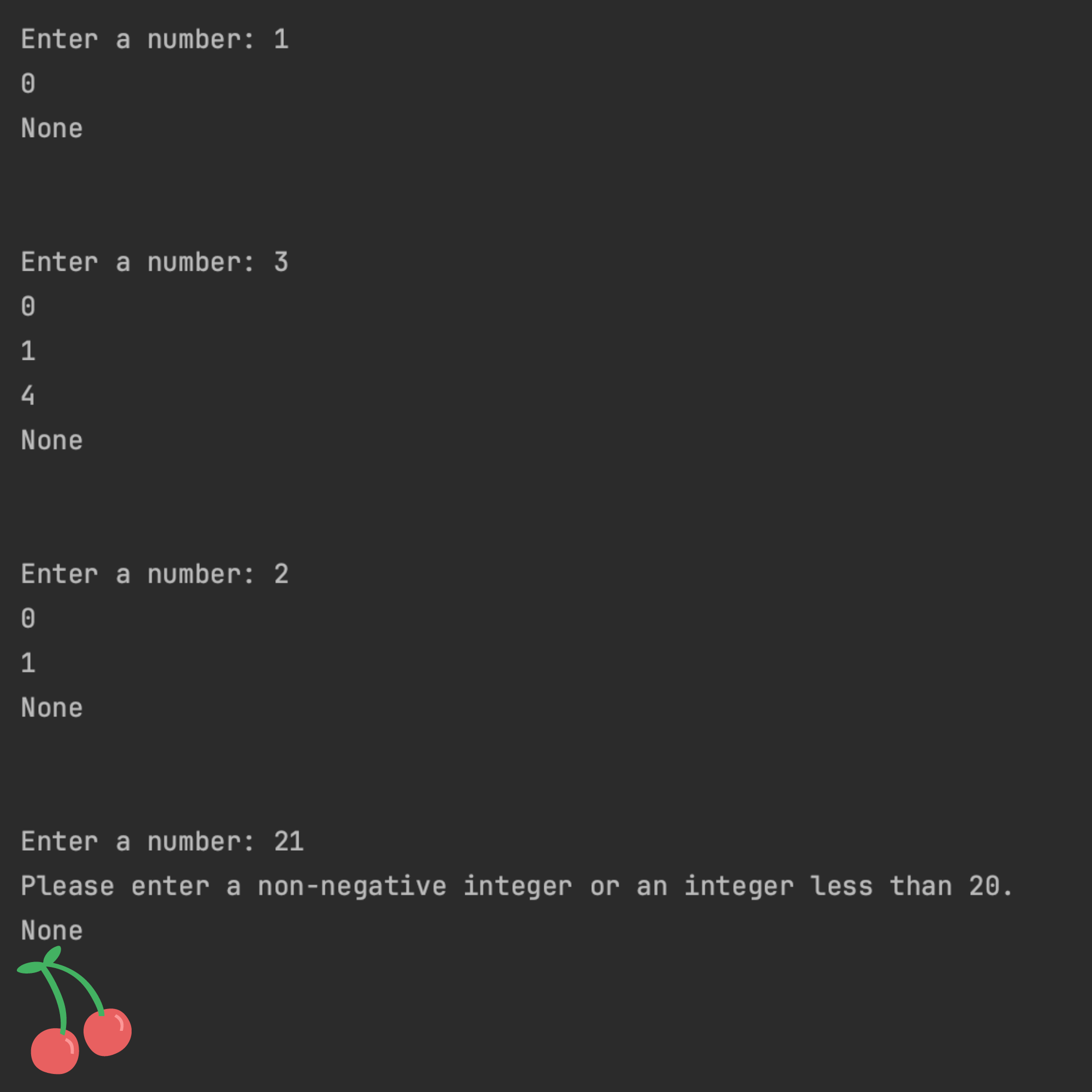
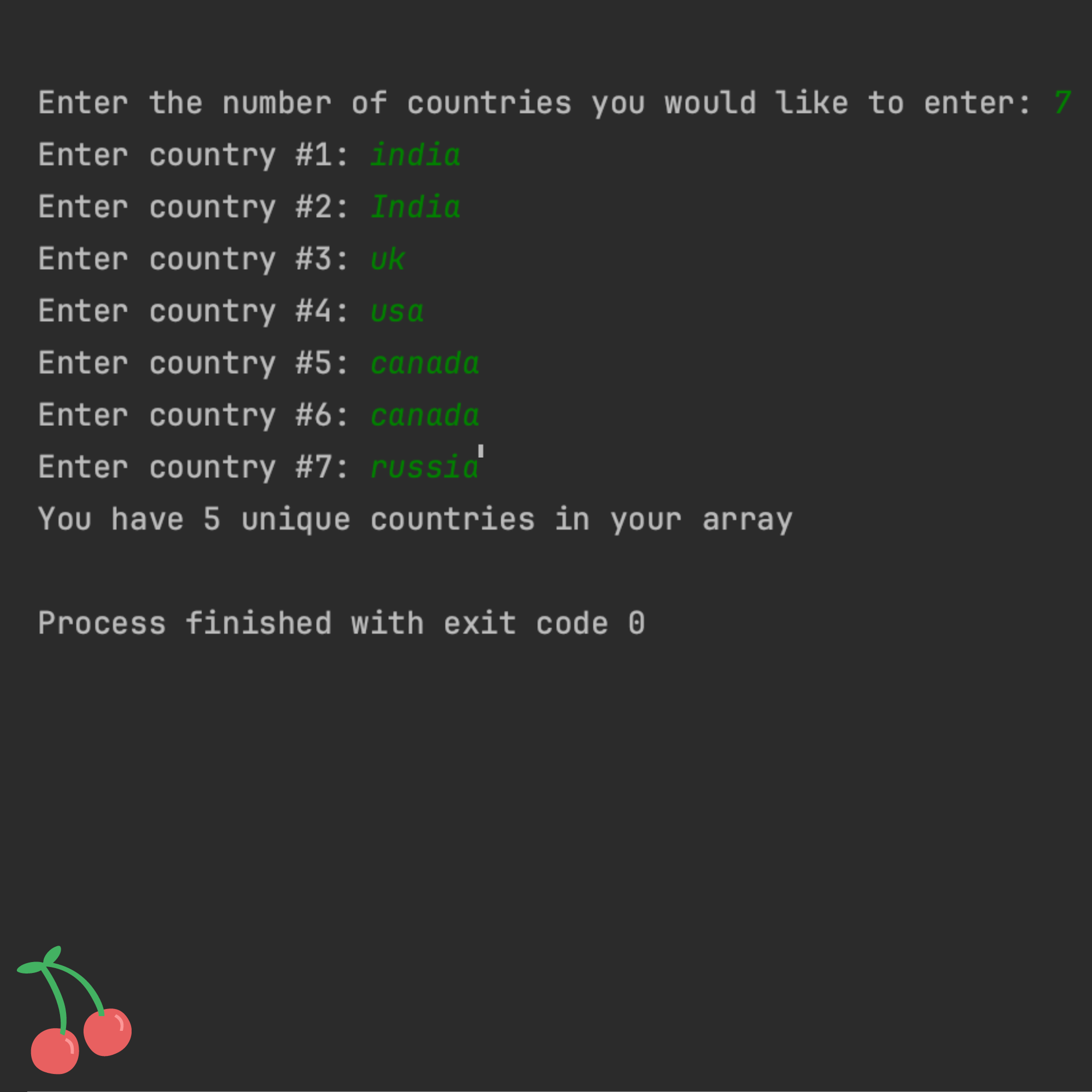
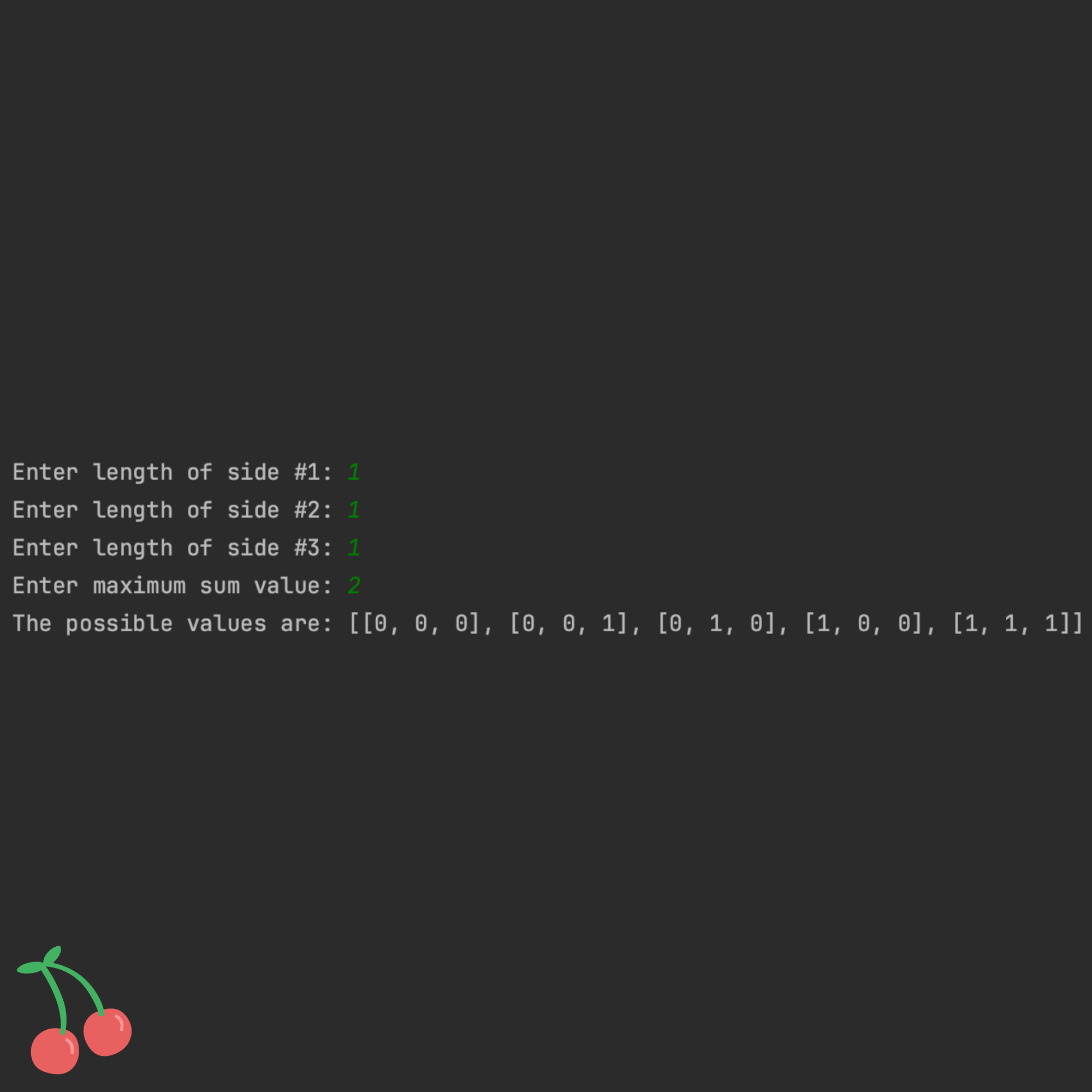
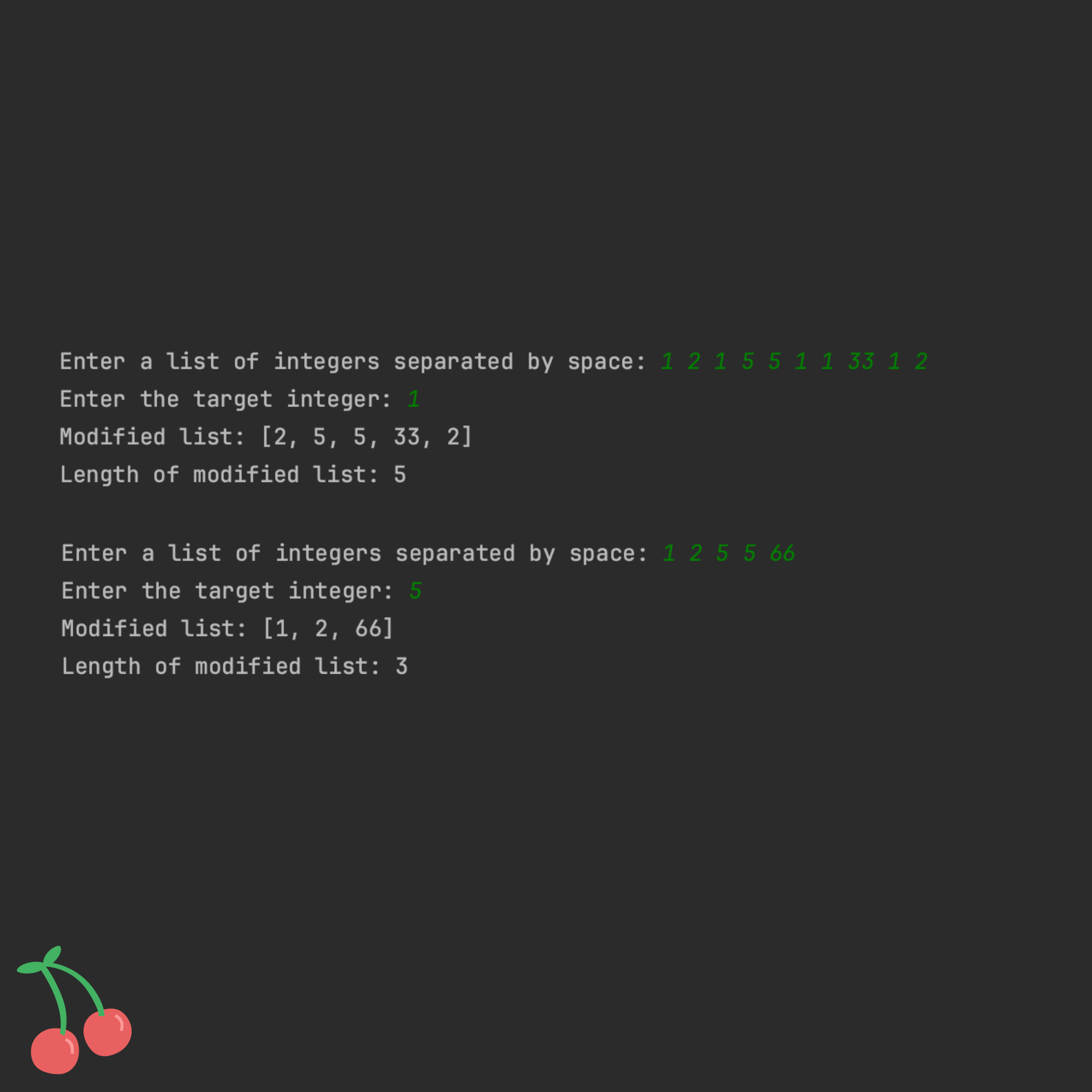
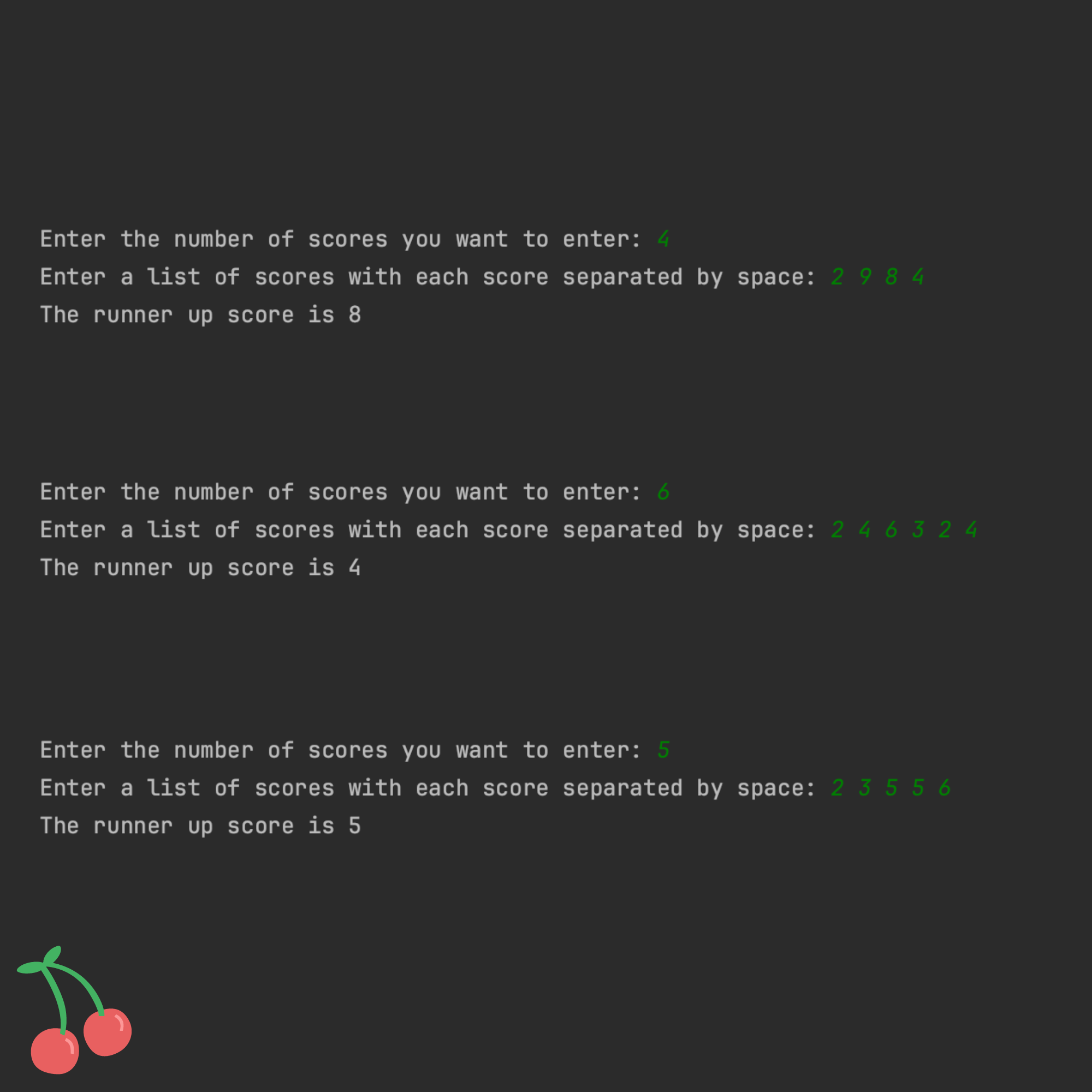
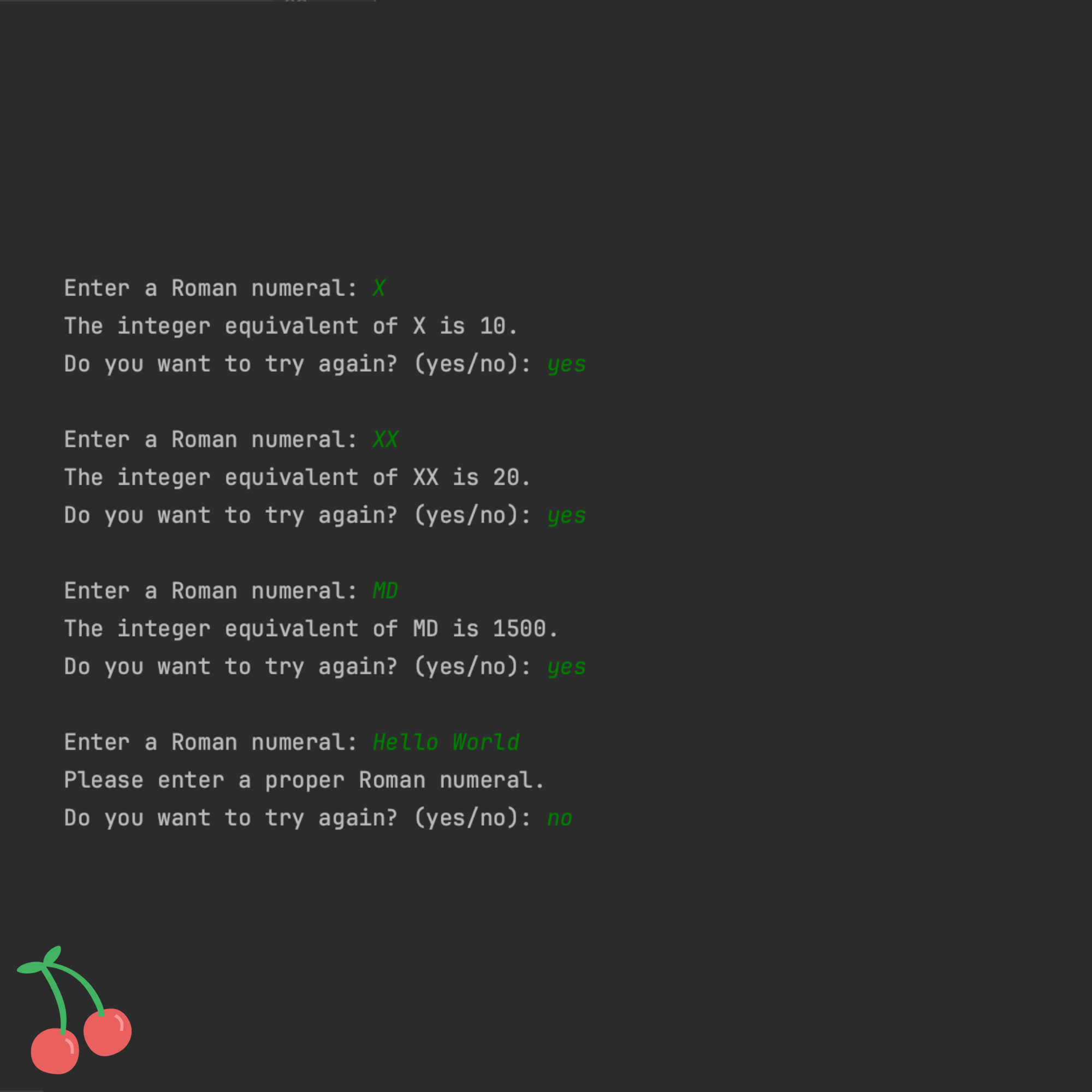
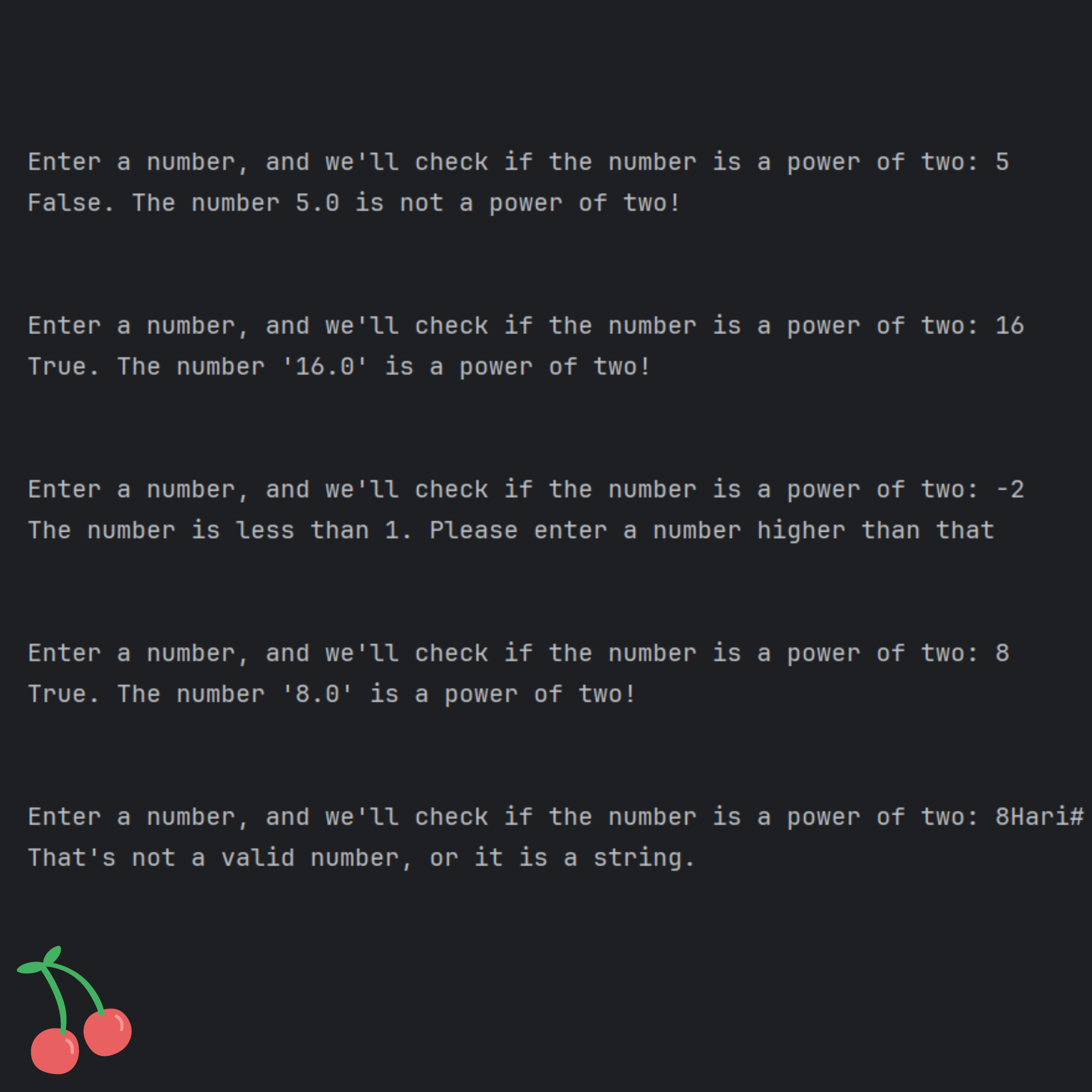
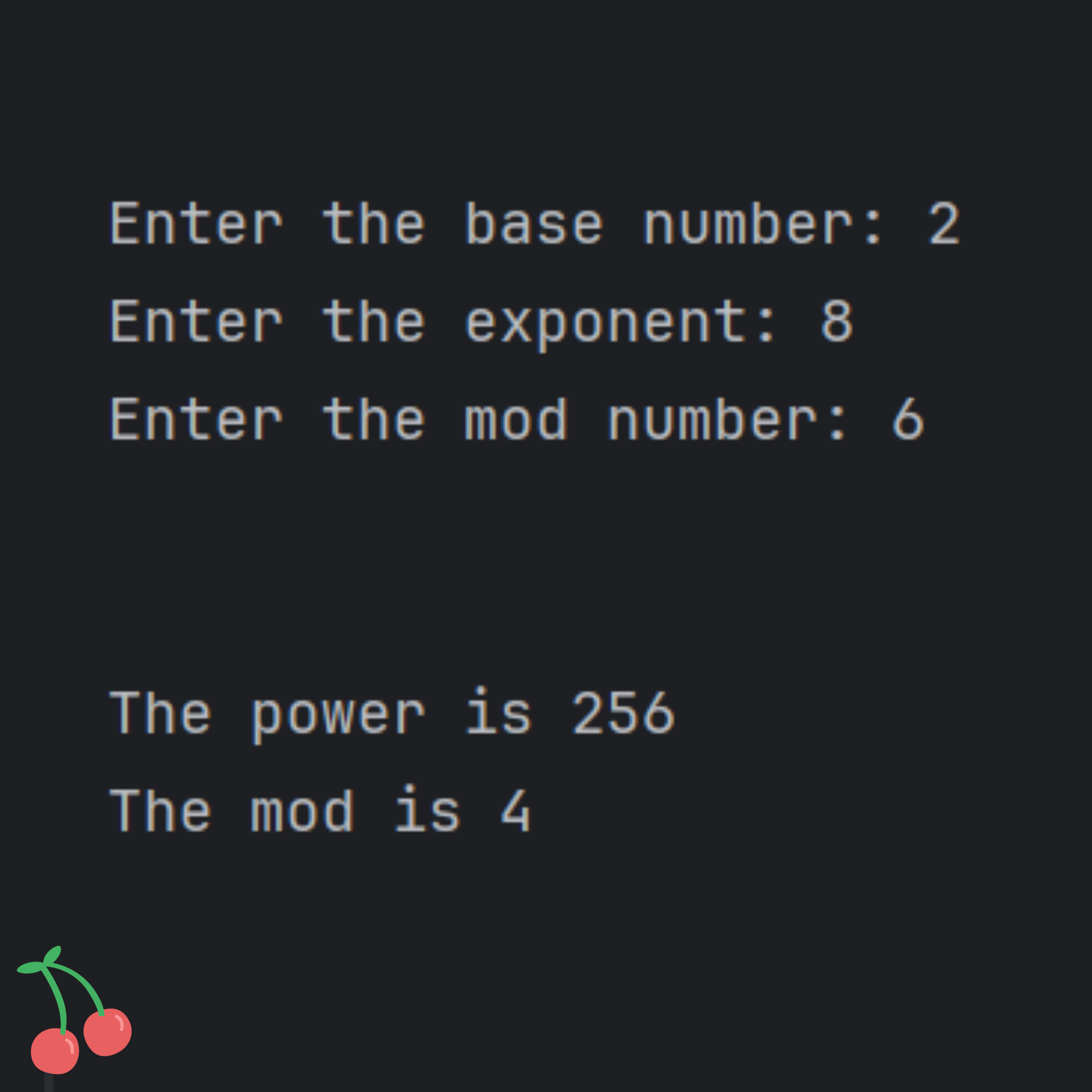
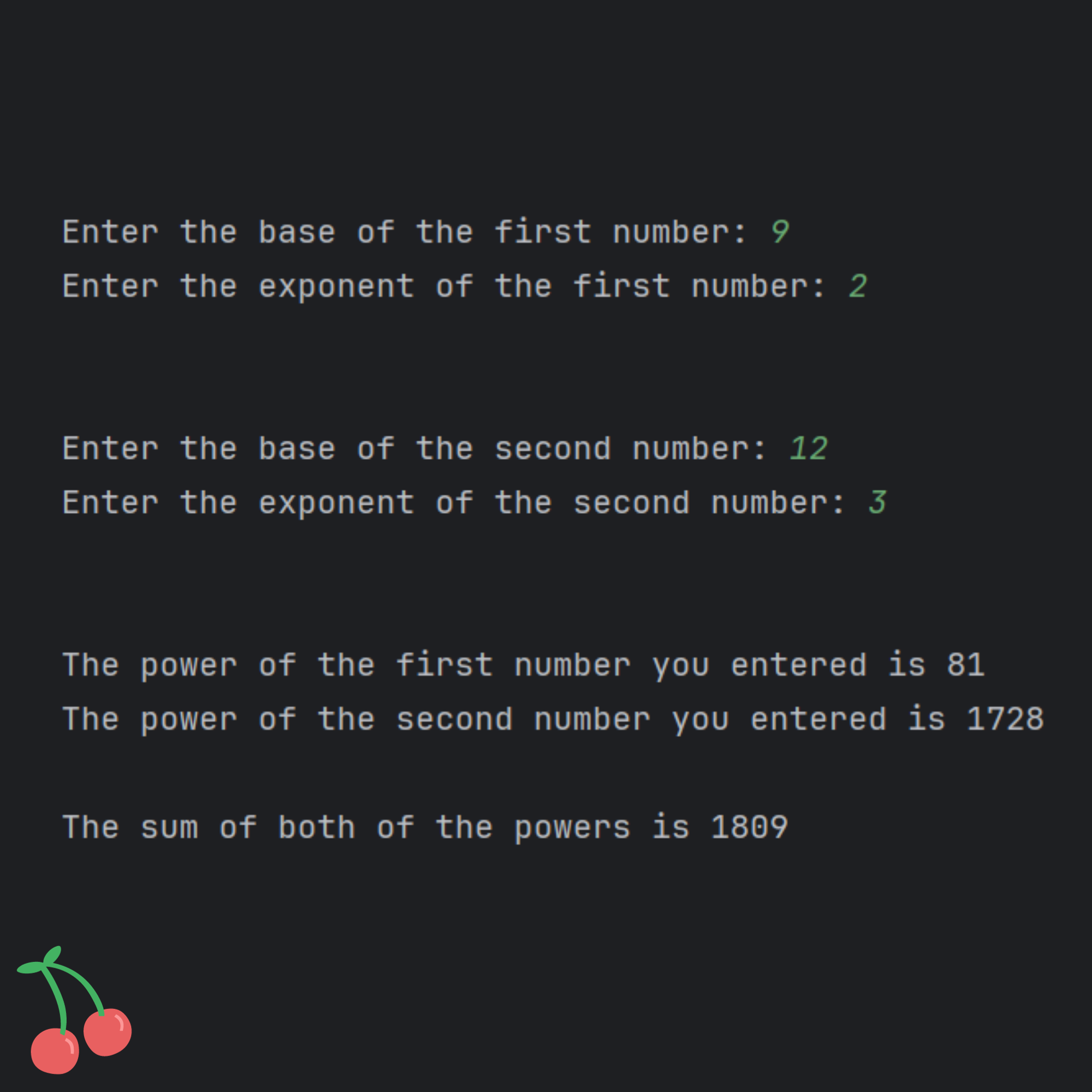
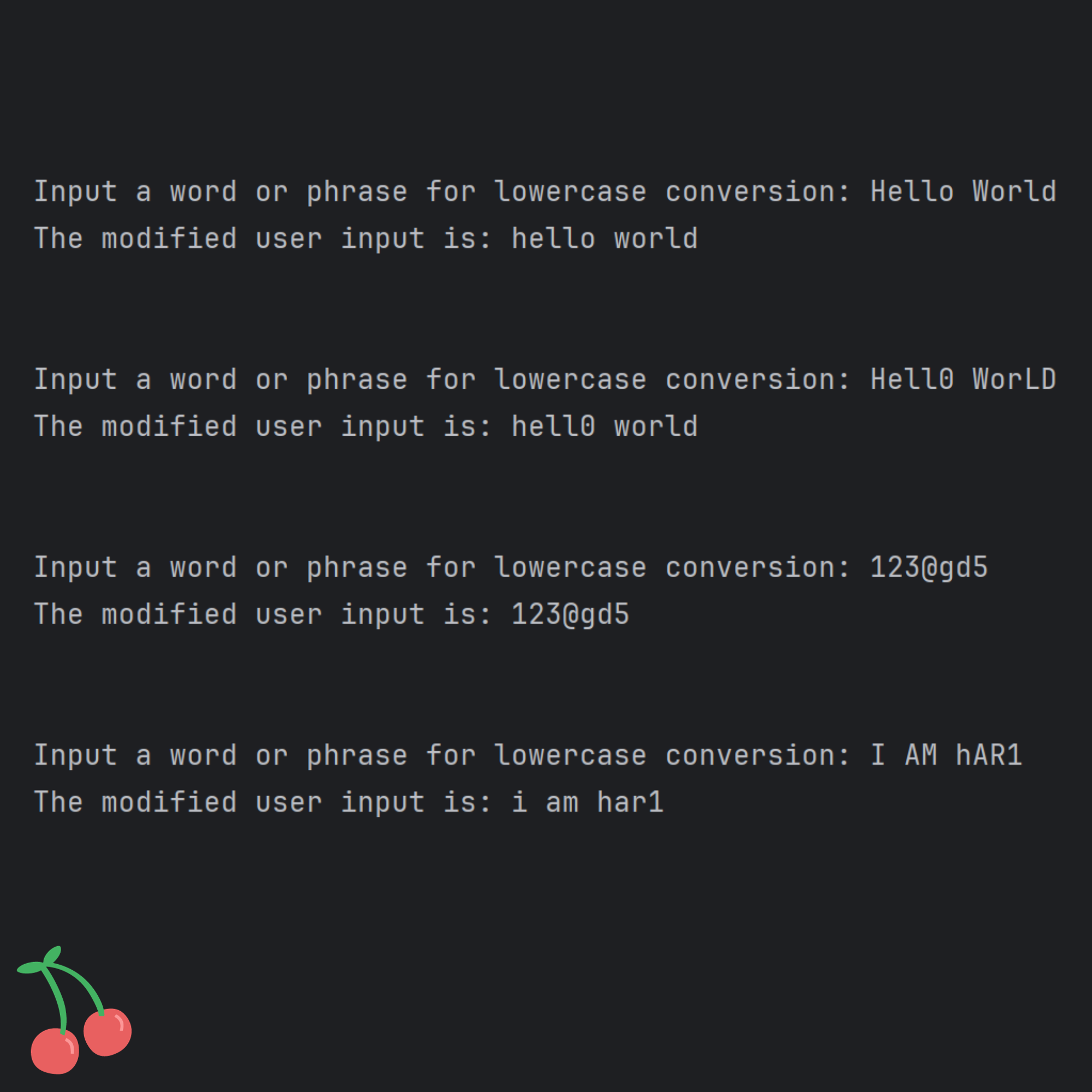
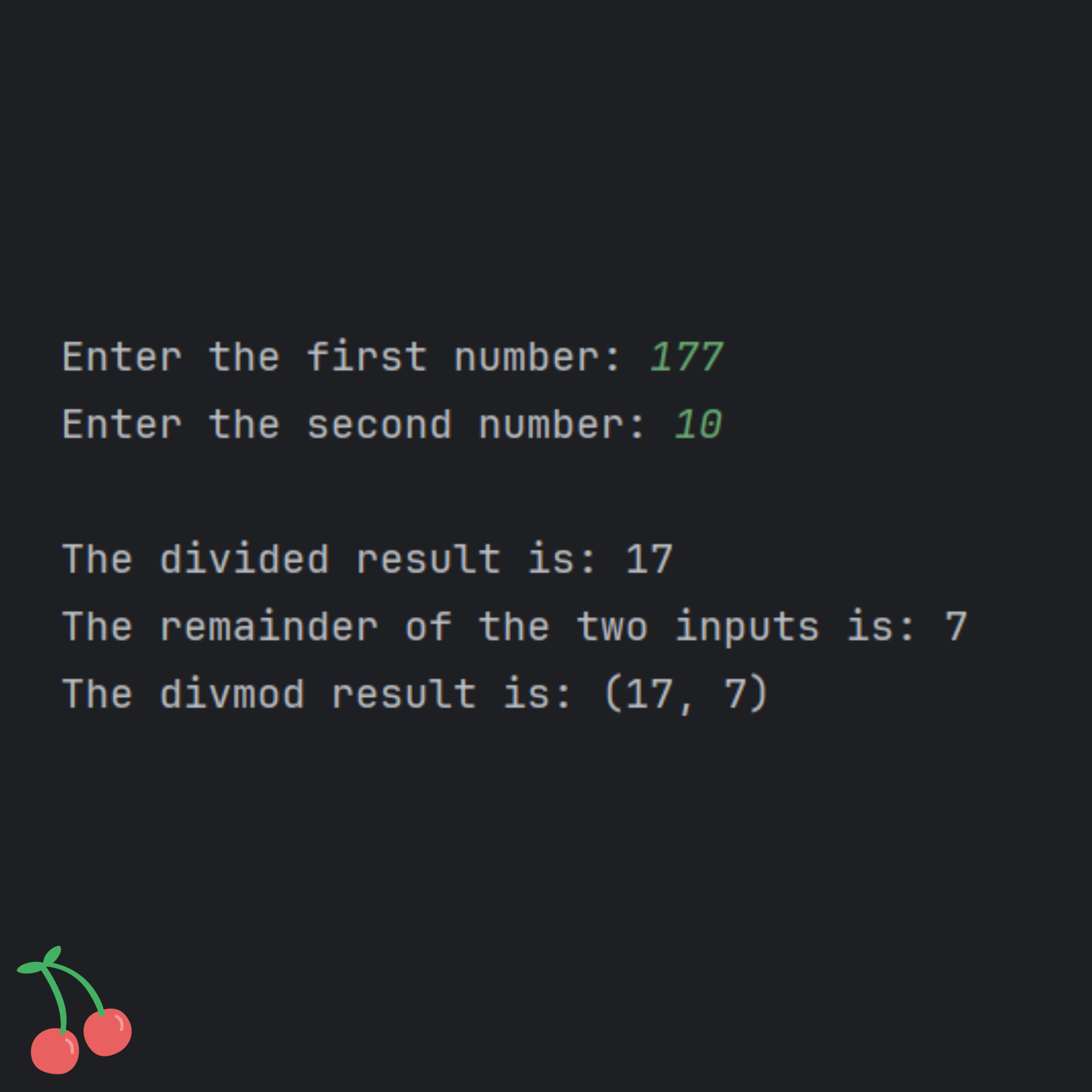
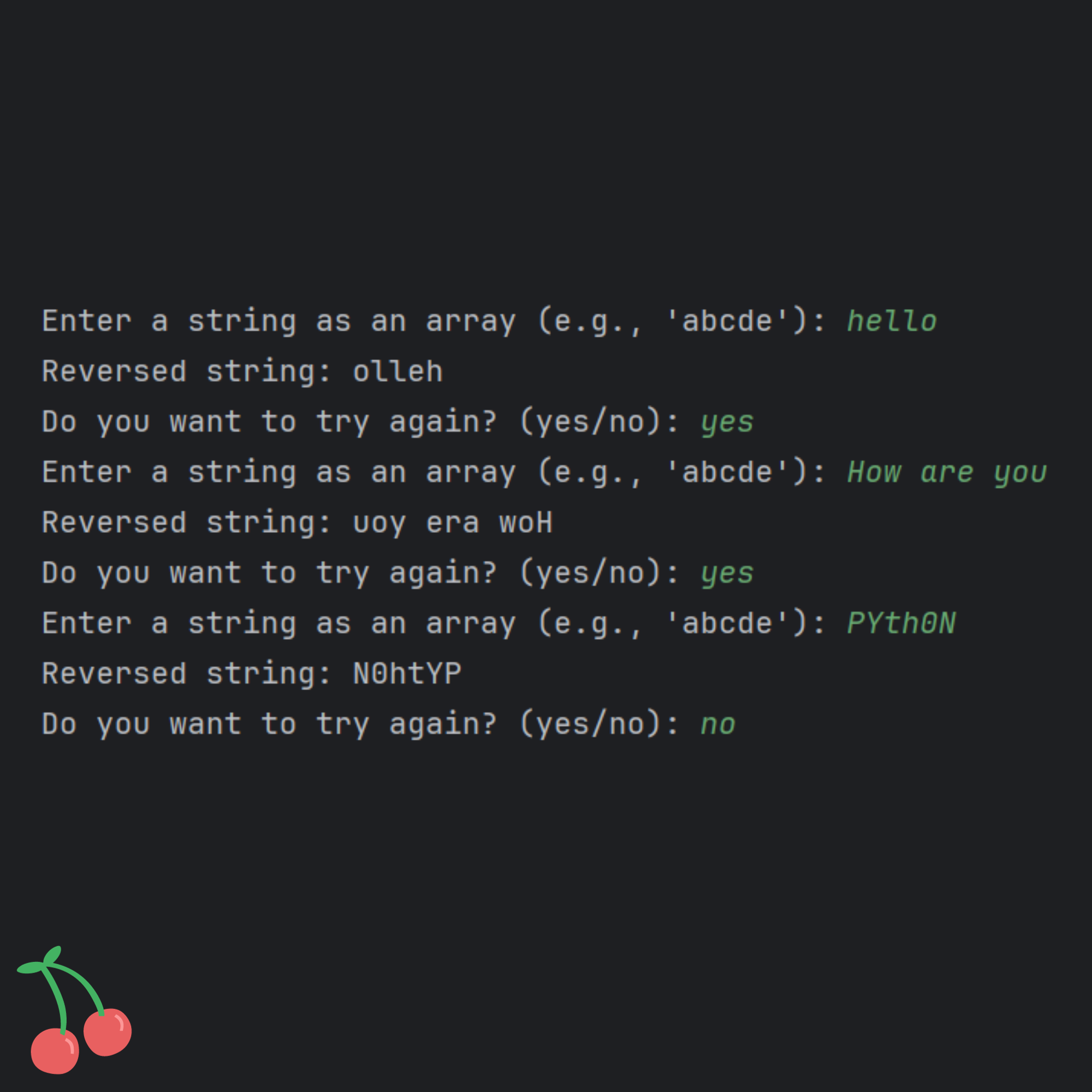
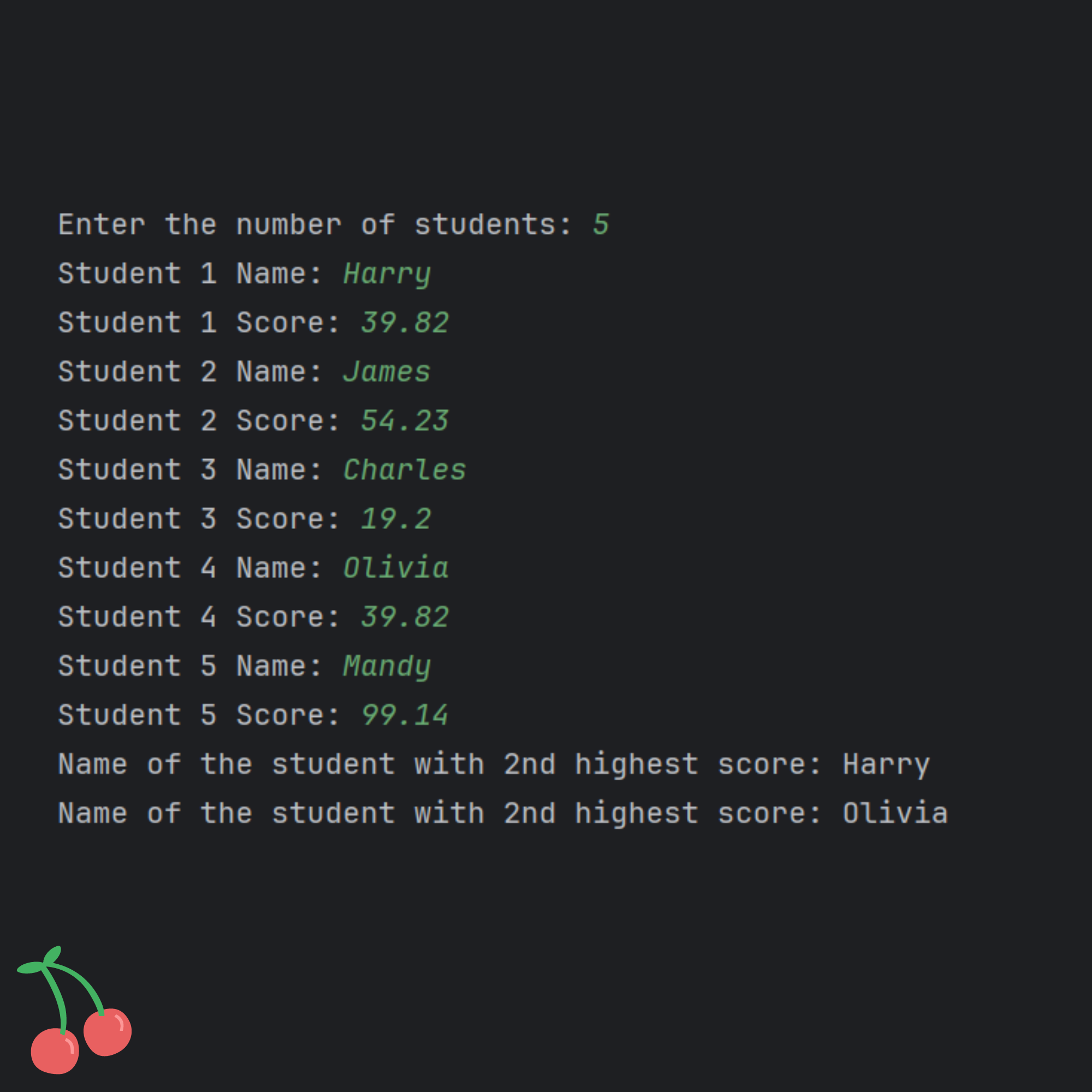
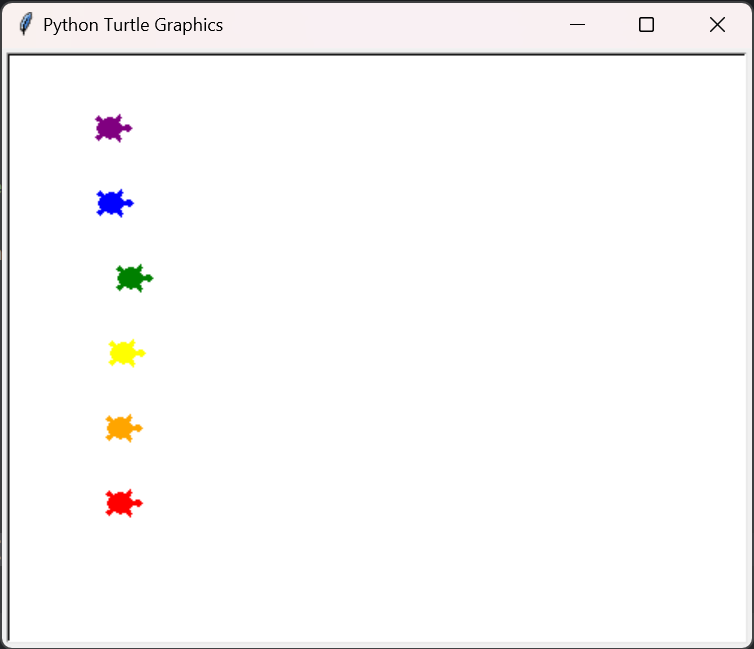
Explore my categorized projects below. The math section includes coding projects like a calculator, and the science section has a density calculator.